Simple Date Validation and Year Completion in JavaScript
Web Design Agency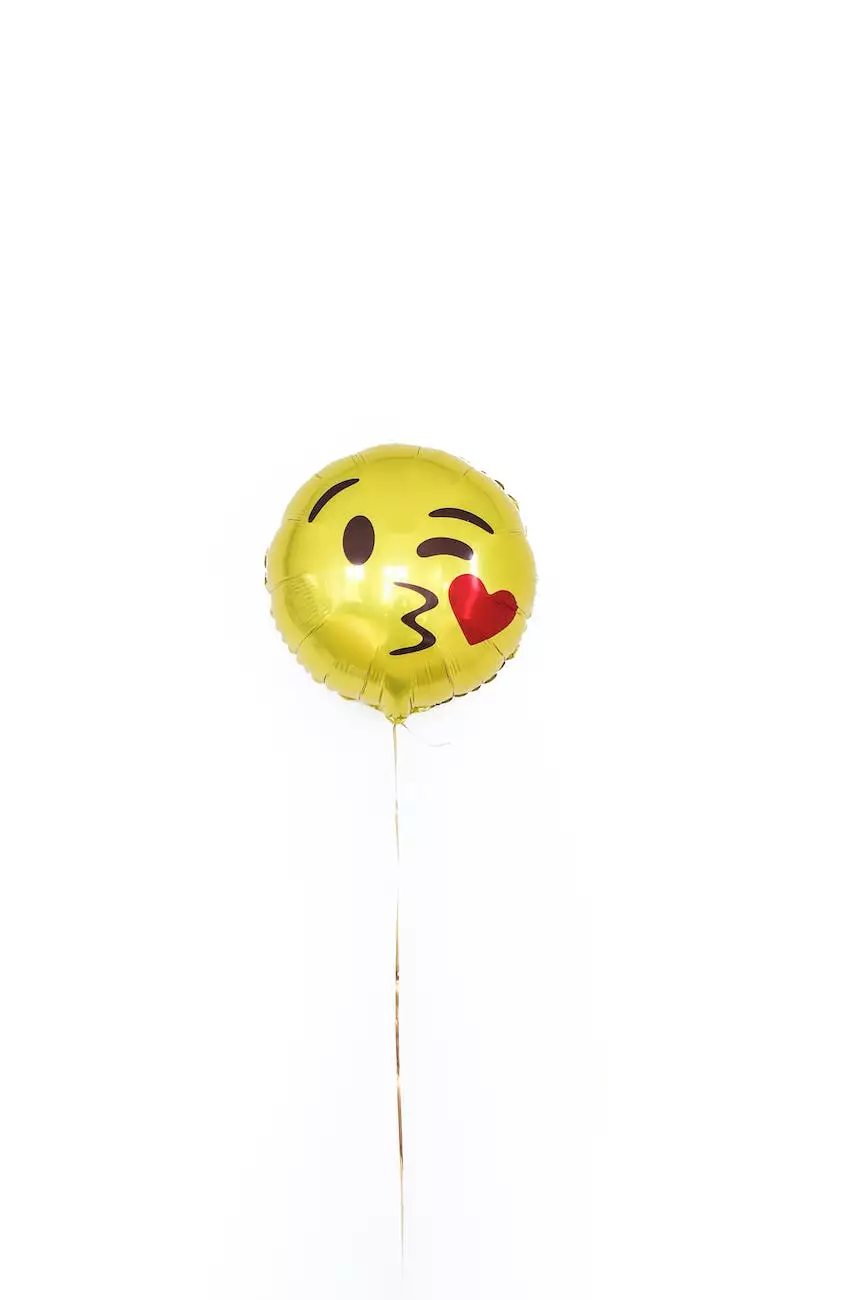
Introduction
Are you looking for a simple way to validate dates and complete missing years in JavaScript? Look no further! Solutions Eighty Seven, a top-notch company specializing in Business and Consumer Services - Digital Marketing, is here to provide you with a comprehensive guide on how to perform simple date validation and year completion using JavaScript.
Why is Date Validation Important?
Date validation is crucial in various scenarios, such as form submissions, data processing, and generating accurate reports. By ensuring that the dates entered are valid, you can prevent errors and maintain data integrity.
How to Validate a Date in JavaScript
JavaScript provides built-in functions and methods to validate dates. One common method is to use regular expressions to match the desired date format. Here's an example:
function validateDate(dateString) { var pattern = /^\d{1,2}\/\d{1,2}\/\d{4}$/; if (!pattern.test(dateString)) { return false; } var dateParts = dateString.split("/"); var day = parseInt(dateParts[0], 10); var month = parseInt(dateParts[1], 10) - 1; var year = parseInt(dateParts[2], 10); var date = new Date(year, month, day); if (date.getFullYear() !== year || date.getMonth() !== month || date.getDate() !== day) { return false; } return true; }In the above code, we define a validateDate function that takes a dateString as input. We use a regular expression pattern to check if the date matches the desired format (DD/MM/YYYY). If it does, we split the string to get the day, month, and year values. We then create a new Date object and compare its parts with the original values to ensure they match.
Completing Missing Years in Dates
What if you receive a date without a specified year? Solutions Eighty Seven has you covered! We've crafted a JavaScript function to help you complete missing years in dates. Take a look at the code snippet below:
function completeYear(dateString, defaultYear) { var dateParts = dateString.split("/"); var day = parseInt(dateParts[0], 10); var month = parseInt(dateParts[1], 10) - 1; var year = dateParts[2] ? parseInt(dateParts[2], 10) : defaultYear; var date = new Date(year, month, day); return date.toLocaleDateString(); }The completeYear function above takes two parameters: dateString and defaultYear. It splits the date string, similar to the previous example, and checks if a year is present. If a year is not specified, it uses the defaultYear value provided. Finally, it creates a new Date object and returns the updated date string with the completed year using the toLocaleDateString method.
Conclusion
Validating dates and completing missing years in JavaScript is essential for maintaining data accuracy and preventing errors. Solutions Eighty Seven, a leading company in Business and Consumer Services - Digital Marketing, has provided you with a comprehensive guide on how to perform these tasks effortlessly using JavaScript.
Remember, ensuring data integrity and accuracy is crucial for your digital marketing efforts. Feel free to reach out to Solutions Eighty Seven's experts for any further assistance or to learn more about our digital marketing services.